Async
async def dependency():
return "Hello, World!"
Sync
def dependency():
return "Hello, World!"
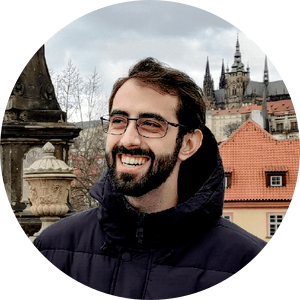
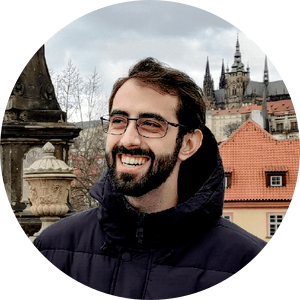
Reference: https://github.com/koddr/starlette-logo
FastAPI is a web framework.
Uvicorn was built by Tom Christie, the same author as
MkDocs, Starlette, HTTPX and Django Rest Framework.
Hypercorn was built by Phil Jones, one of the Flask
maintainers.
Granian was built by an Italian - which is in the room.
The client initiates the communication, and the server
parses the HTTP request. Then the server sends the information
to the application in a specific format.
ASGI is the interface between the server and the application.
And the request line is read...
The middleware intercepts the messages sent by the server, and the application.
When to use non-async code?
- CPU bound that can escape the GIL
- Thread blocking IO